Generate a CSV file from data using Node.js
Exporting data from the application can be helpful for many use cases like sharing data between applications, making business decisions, etc...
CSV, which stands for Comma Separated Value, is a file type to store the data. The format is simple, easy to read data from, and many editors can open it. In this project, we will see how to create a CSV file and write data inside.
If you are interested in how to read data in CSV file, I wrote a whole post about:
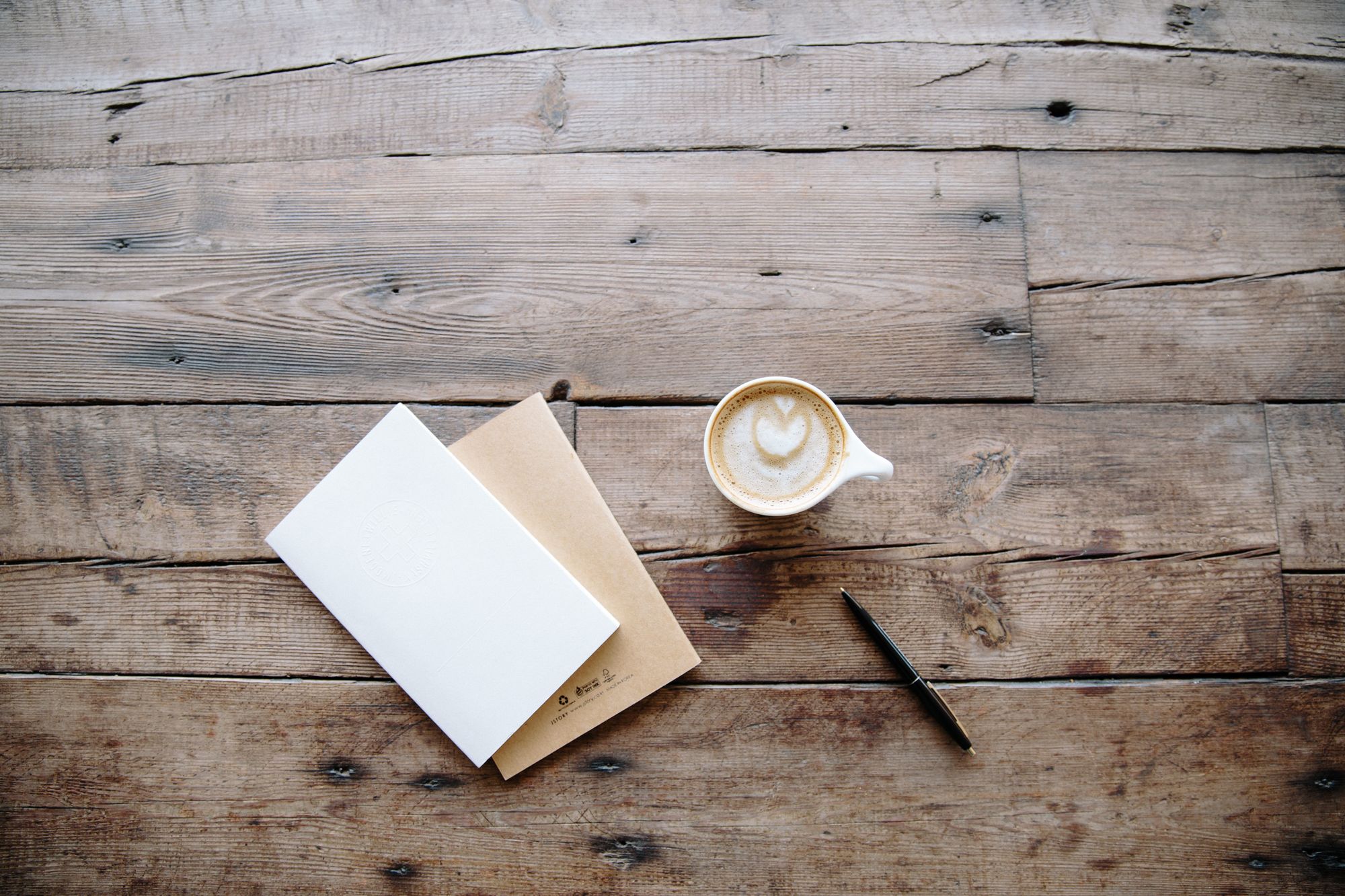
Set up the project
Make sure you have Node.js installed on your computer before continuing. I prepared a Node.js project starter with Typescript. Let's clone it and make it work.
git clone https://github.com/tericcabrel/node-ts-starter node-csv-write
cd node-csv-write
yarn install # or npm install
yarn start # or npm run start
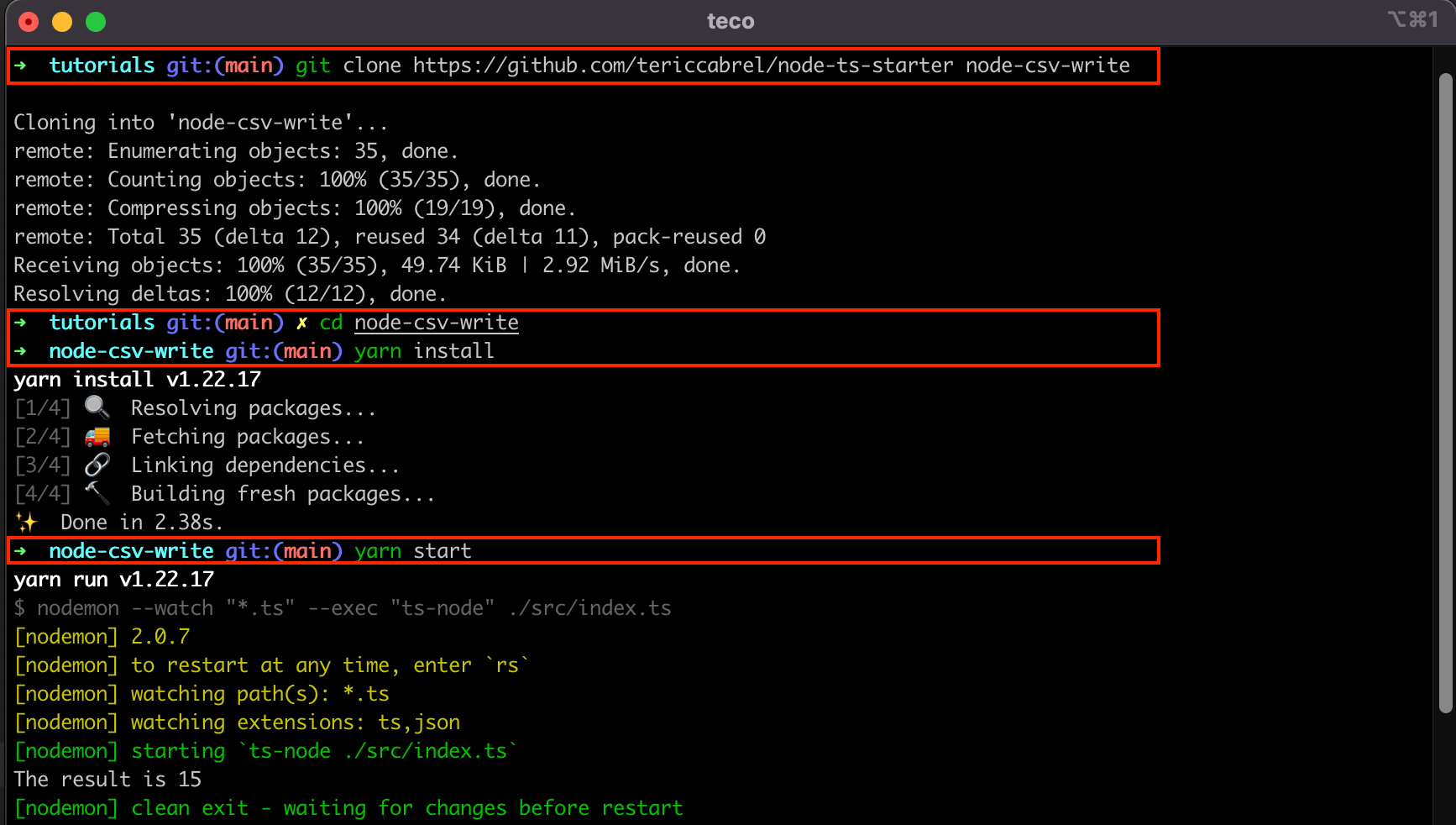
Define the data to write
Let's say we retrieve a list of countries from the database, and we want to write them in a CSV file. The first is to define the sample of data we want to write.
Delete the content on the file src/index.ts
and add the code below:
type Country = {
name: string;
countryCode: string;
capital: string;
phoneIndicator: number;
};
const countries: Country[] = [
{ name: 'Cameroon', capital: 'Yaounde', countryCode: 'CM', phoneIndicator: 237 },
{ name: 'France', capital: 'Paris', countryCode: 'FR', phoneIndicator: 33 },
{ name: 'United States', capital: 'Washington, D.C.', countryCode: 'US', phoneIndicator: 1 },
{ name: 'India', capital: 'New Delhi', countryCode: 'IN', phoneIndicator: 91 },
{ name: 'Brazil', capital: 'Brasília', countryCode: 'BR', phoneIndicator: 55 },
{ name: 'Japan', capital: 'Tokyo', countryCode: 'JP', phoneIndicator: 81 },
{ name: 'Australia', capital: 'Canberra', countryCode: 'AUS', phoneIndicator: 61 },
{ name: 'Nigeria', capital: 'Abuja', countryCode: 'NG', phoneIndicator: 234 },
{ name: 'Germany', capital: 'Berlin', countryCode: 'DE', phoneIndicator: 49 },
];
Note: I get the information about countries here: https://restcountries.com/
Install the package csv-writer
csv-writer is the package we will use to create the csv file and add the data inside the file.
Let's install it:
yarn add csv-writer
Define the columns' header
A CSV has two main parts: the header and data rows. To create the header, we need to map each column of the header to a property of the Country object.
In the file src/index.ts
, below is the code to initialize the csv writer:
import * as path from 'path';
const csvWriter = require('csv-writer');
const writer = csvWriter.createObjectCsvWriter({
path: path.resolve(__dirname, 'countries.csv'),
header: [
{ id: 'name', title: 'Name' },
{ id: 'countryCode', title: 'Country Code' },
{ id: 'capital', title: 'Capital' },
{ id: 'phoneIndicator', title: 'International Direct Dialling' },
],
});
The property path holds the path where to store the file created, and the property header has an array of items where each item represents a column of the CSV.
The value of the property ID must be a key of the object Country (name
, countryCode
, capital
and phoneIndicator
). The property title can be anything and will be displayed as the header in the CSV file.
Write the data to the file
Here is the final content of the file src/index.ts
import * as path from 'path';
const csvWriter = require('csv-writer');
type Country = {
name: string;
countryCode: string;
capital: string;
phoneIndicator: number;
};
const countries: Country[] = [
{ name: 'Cameroon', capital: 'Yaounde', countryCode: 'CM', phoneIndicator: 237 },
{ name: 'France', capital: 'Paris', countryCode: 'FR', phoneIndicator: 33 },
{ name: 'United States', capital: 'Washington, D.C.', countryCode: 'US', phoneIndicator: 1 },
{ name: 'India', capital: 'New Delhi', countryCode: 'IN', phoneIndicator: 91 },
{ name: 'Brazil', capital: 'Brasília', countryCode: 'BR', phoneIndicator: 55 },
{ name: 'Japan', capital: 'Tokyo', countryCode: 'JP', phoneIndicator: 81 },
{ name: 'Australia', capital: 'Canberra', countryCode: 'AUS', phoneIndicator: 61 },
{ name: 'Nigeria', capital: 'Abuja', countryCode: 'NG', phoneIndicator: 234 },
{ name: 'Germany', capital: 'Berlin', countryCode: 'DE', phoneIndicator: 49 },
];
const writer = csvWriter.createObjectCsvWriter({
path: path.resolve(__dirname, 'countries.csv'),
header: [
{ id: 'name', title: 'Name' },
{ id: 'countryCode', title: 'Country Code' },
{ id: 'capital', title: 'Capital' },
{ id: 'phoneIndicator', title: 'International Direct Dialling' },
],
});
writer.writeRecords(countries).then(() => {
console.log('Done!');
});
Execute the file with the command: yarn start
.
Verify everything works as expected
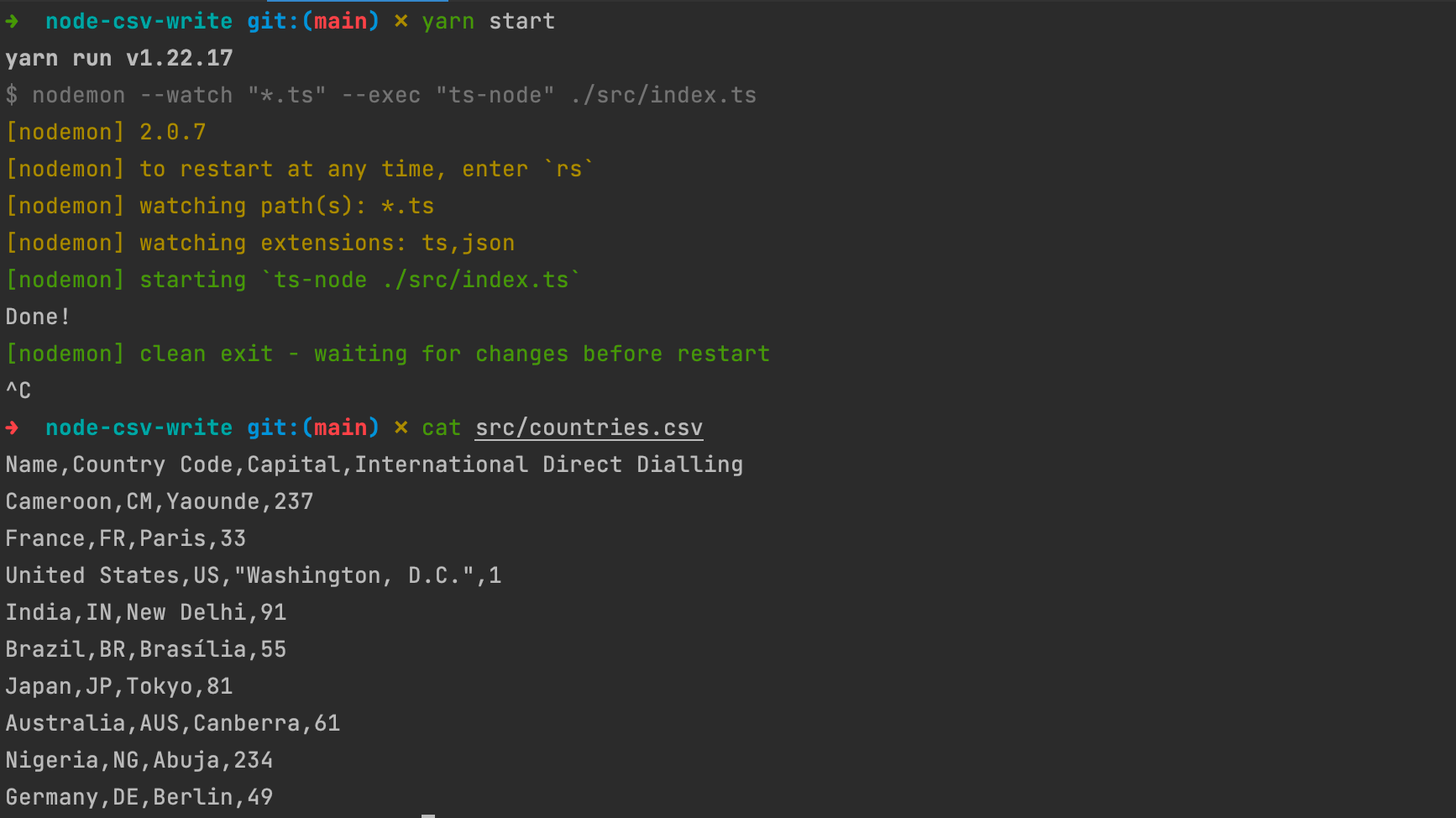
Check out the package documentation to learn about other options.
You can find the code source on the GitHub repository.
Follow me on Twitter or subscribe to my newsletter to avoid missing the upcoming posts and the tips and tricks I occasionally share.