Install and use the AWS SDK v3 in Node.js
In December 2020, AWS announced the general availability of the AWS SDK v3, which improved the way developers build and deploy applications that interact with AWS services using the SDK.
Almost four years ago, the AWS SDK v2 was still widely used, with almost 10 million downloads per week (if the value is incorrect, it is because of developers that migrated after reading my post 😌); developers could benefit from a better developer experience and application performance improvements by migrating to v3.
This post will show how to install and use the AWS SDK v3 for Node.js to interact with an AWS service.
Why you should use the AWS SDK v3
The AWS SDK v3 comes with major improvements in comparison to the v2 that we can enumerate the following:
- Modular package: Instead of publishing a single package containing all the functions for all the services, each service is now published as a Node.js package under the scope
@aws-sdk
. This reduces the bundle size, which is essential for platforms such as Mobile, Lambda Function, IoT, etc... - TypeScript support: You have a better developer experience by having typing and autocomplete and the static and runtime type check, preventing you from deploying non-functioning code in production.
- Middleware stack: allows you to easily customize SDK behavior by adding more information in the execution context; you can add your custom asynchronous actions and/or remove default ones.
- Active maintenance: The AWS SDK v3 is actively maintained while the v2 is still maintained but is entering the non-maintenance mode.
- Securities patches: By being actively maintained, you will gain feature updates and also critical securities fixes and patches, which are essential for a client interacting with your services in production.
Install AWS SDK v3
The major difference with the AWS SDK v2 is that instead of installing a single package containing all the services SDK, you can install a Node.js package only for the service you need.
The package's pattern is @aws-sdk/client-<service-name>
You must refer to the AWS SDK for JavaScript V3 API Reference Guide to find the AWS service's name, which is in the left sidebar under the section "services".
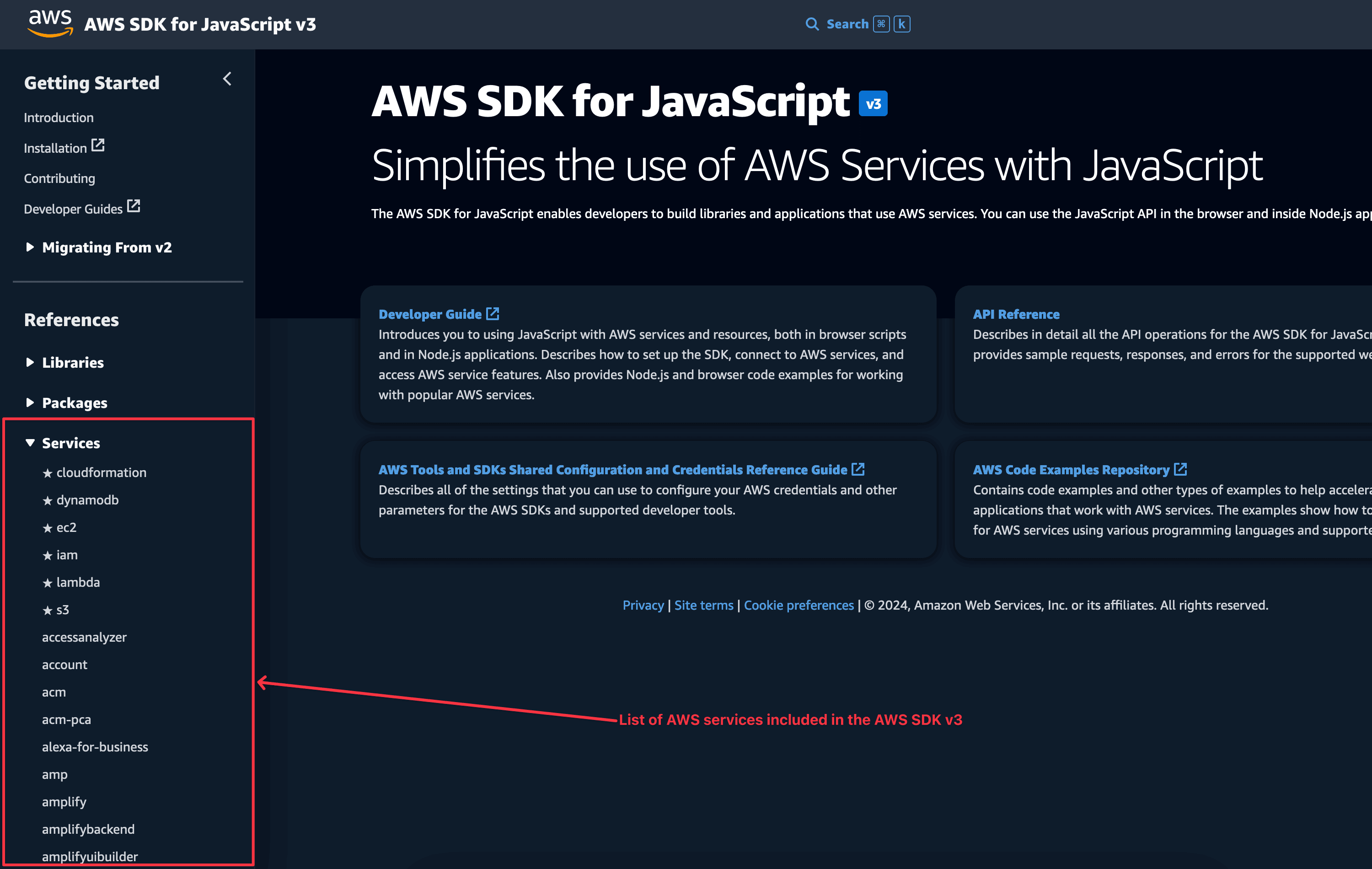
Let's say you are building a backend REST API and need the SDK to interact with the following services: AWS Lambda, S3, CloudFront, SNS, SQS, and API Gateway.
Here is the command to install these packages:
npm install @aws-sdk/client-lambda \
@aws-sdk/client-s3 \
@aws-sdk/client-cloudfront \
@aws-sdk/client-sns \
@aws-sdk/client-sqs
With Yarn, it will be
yarn add @aws-sdk/client-lambda \
@aws-sdk/client-s3 \
@aws-sdk/client-cloudfront \
@aws-sdk/client-sns \
@aws-sdk/client-sqs
That is it!!! After, you must read the API reference for each service to see which methods are available and how to use them.
- API reference for AWS Lambda client SDK v3
- API reference for AWS S3 client SDK v3
- API reference for AWS CloudFront client SDK v3
- API reference for AWS SNS client SDK v3
- API reference for AWS SQS client SDK v3
Demo with the AWS Lambda SDK client
Let's say you have your backend API, and you want to programmatically invoke a Lambda function and get the returned result. Let's see how to do that with the AWS SDK client v3
Prerequisites
To follow this tutorial, make sure you have the following tools installed on your computer.
- An AWS account with a free tier; create an account here.
- Node.js 18+ and Yarn - Download link
- The AWS credentials to authenticate. Read my blog post to generate one.
- An AWS Lambda Function deployed. Read my blog post to quickly deploy one.
Set up the project
We will use the starter template we built in this tutorial to quickly bootstrap a Node.js project with TypeScript.
Run the command below to set up the project:
git clone https://github.com/tericcabrel/node-ts-starter.git node-lambda-sdk
cd node-lambda-sdk
yarn install
yarn start
The last command will execute the project and print the outer in the console
Install the AWS Lambda SDK client v3
As we saw earlier, run this command to install the SDK
yarn add @aws-sdk/client-lambda
The Lambda SDK client requires the AWS secret key ID and the AWS secret access key to authenticate. We will read these values from the environment variables file with the help of the Node.js package dotenv.
yarn add dotenv
Create a file name .env
and add the code below:
AWS_ACCESS_KEY_ID=<value>
AWS_SECRET_ACCESS_KEY=<value>
Replace the <value>
with your AWS credentials.
Invoke a Lambda Function with the AWS SDK client v3
Now we can use it to invoke the Lambda Function, replace the code of the file src/index.ts
with the code below:
import { configDotenv } from 'dotenv';
import { LambdaClient, InvokeCommand, InvokeCommandInput } from '@aws-sdk/client-lambda';
configDotenv();
const lambdaClient = new LambdaClient({
credentials: {
accessKeyId: process.env.AWS_ACCESS_KEY_ID,
secretAccessKey: process.env.AWS_SECRET_ACCESS_KEY,
},
});
const main = async () => {
const payload = {
body: JSON.stringify({
height: 181,
weight: 88,
}),
};
const input: InvokeCommandInput = {
FunctionName: 'bmi-calculator',
InvocationType: 'RequestResponse',
Payload: JSON.stringify(payload),
};
try {
const command = new InvokeCommand(input);
const response = await lambdaClient.send(command);
const responseString = response.Payload?.transformToString('utf-8') ?? '{}';
console.log(JSON.parse(responseString));
} catch (e) {
console.error(e);
}
};
void main();
We create an instance of the AWS Lambda SDK client with the AWS credentials and prepare the input for the command that invokes the function.
We pass the function name and indicate that we want a synchronous invocation by setting the invocation type to RequestResponse
.
We invoke the function, parse the response, extract the function result, and print it in the console. The execution is wrapped in a try-catch block.
Run the command below to invoke the Lambda function:
yarn start
You will get an output similar to this one:
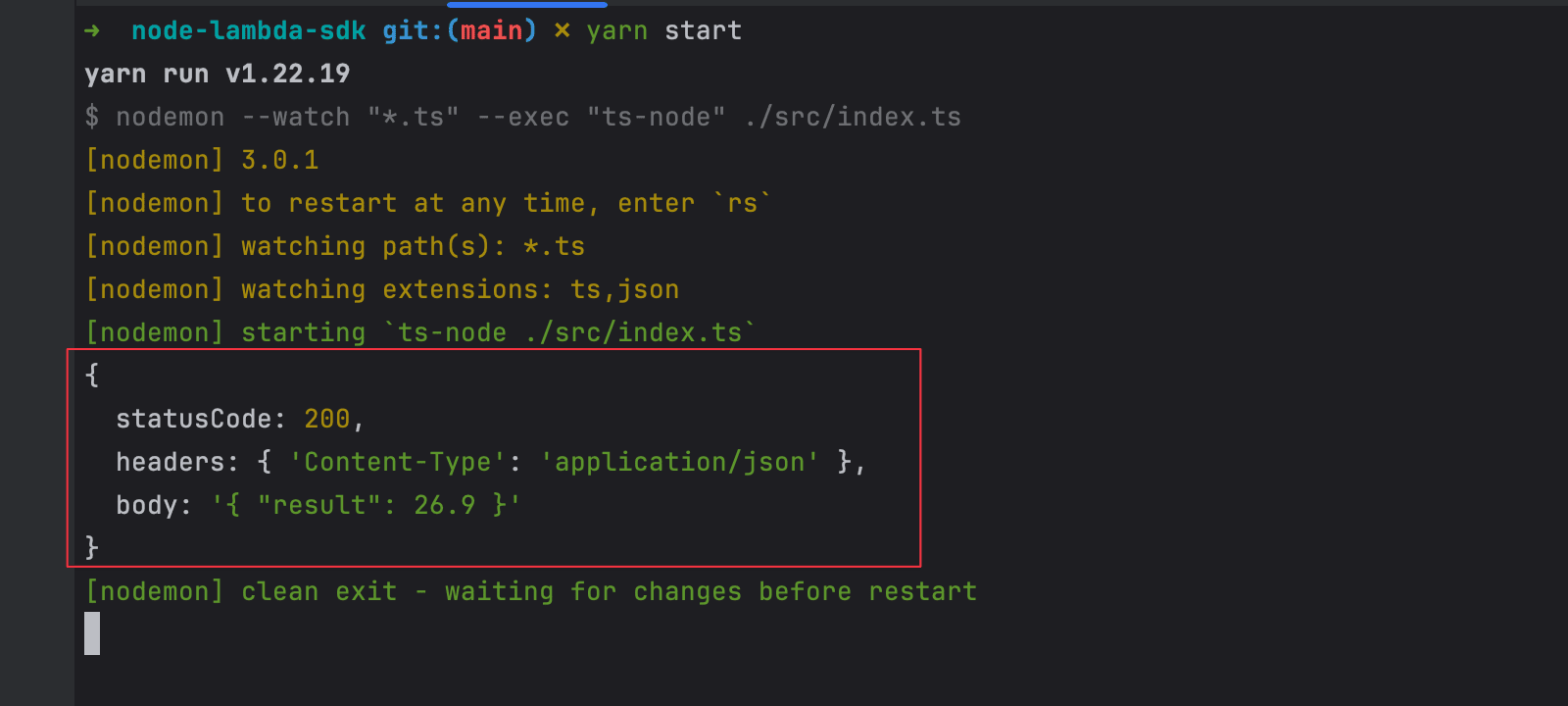
Wrap up
By using the AWS SDKv3, you will gain a better developer experience while building features and improve performance in production, thanks to the reduced bundle size.
For those having a project using the AWS SDK v2 and wanting to migrate to the AWS SDK v3, follow the migration guide.
You can find the code source on the GitHub repository.
Follow me on Twitter or subscribe to my newsletter to avoid missing the upcoming posts and the tips and tricks I occasionally share.