Backup and Restore a MongoDB database
The MongoDB Database Tools is a collection of command-line utilities for working with a MongoDB deployment, including data backup and import/export tools like mongoimport
and mongodump
as well as monitoring tools like mongotop
.
There are many cases where you need to backup and restore your data:
- For security in case of data loss, having a backup help recover faster.
- Replicate the database in a development environment.
- Export a MongoDB collection to use the data as test fixtures.
- Facilitation of data migration or transfer to a new system.
Prerequisites
To follow this post, you will need a MongoDB database having the data you want to back up. You can create a database in the cloud on MongoDB Atlas and use this post I wrote to populate your database.
Install MongoDB database tools
MongoDB provides tools to easily back up and restore the content of your MongoDB database; you must install them on the computer you will perform these operations.
Before version 4.4 of the MongoDB server, the installation automatically included the database tools. Still, the database tools are released separately with their own versioning, so it is okay to have MongoDB 6.0 and the Mongo database tools 100.6.0.
Go to this link to download the MongoDB database tools and select the OS platform to install. No need to change the version to download and package.
I will back up and restore a MongoDB on MacOS, so I choose the "macOS x86_64" platform instead of "macOS arm64" because of my CPU architecture.
Click on the "Download" button.
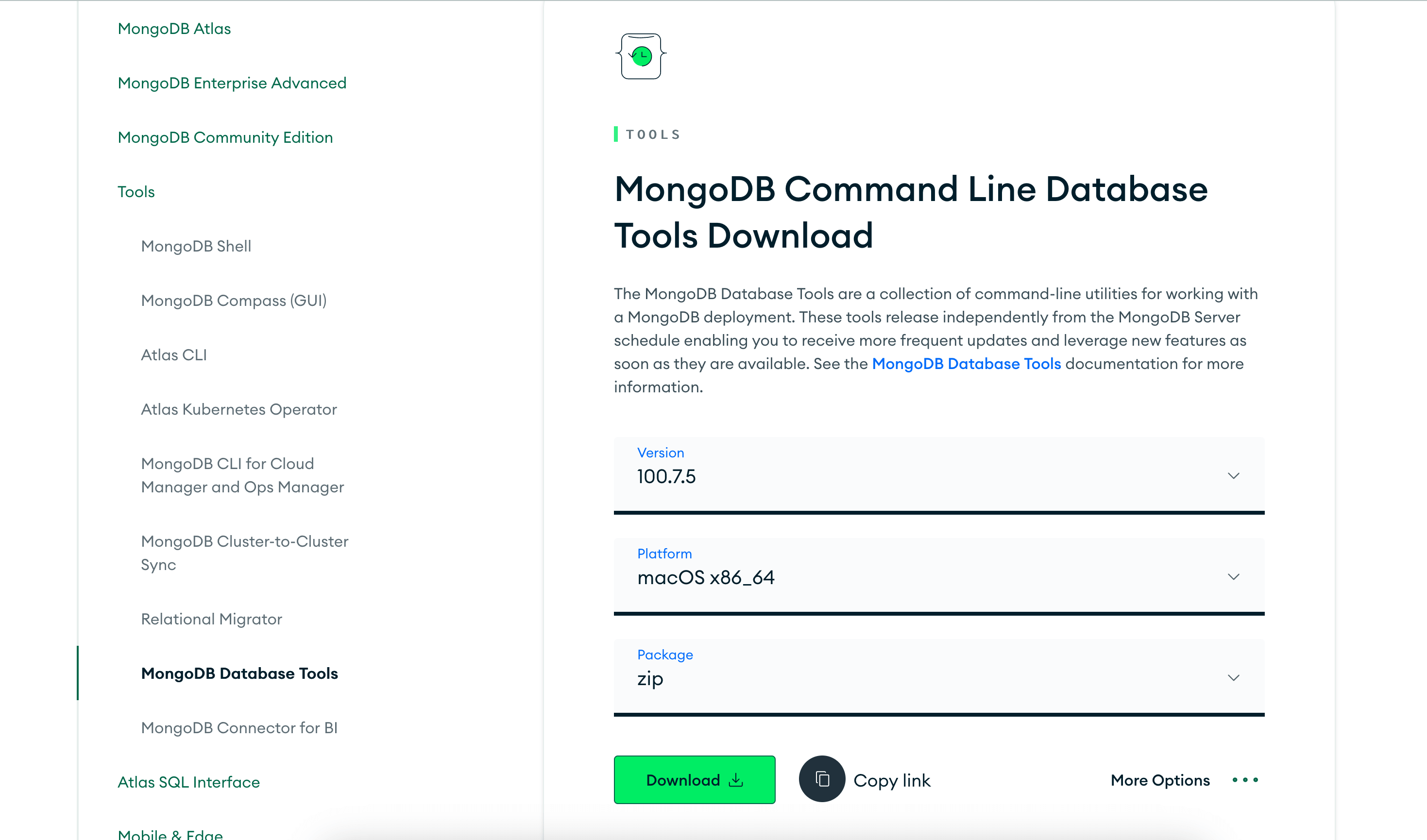
Unzip the folder, and you will find a "bin" folder containing the MongoDB tools.
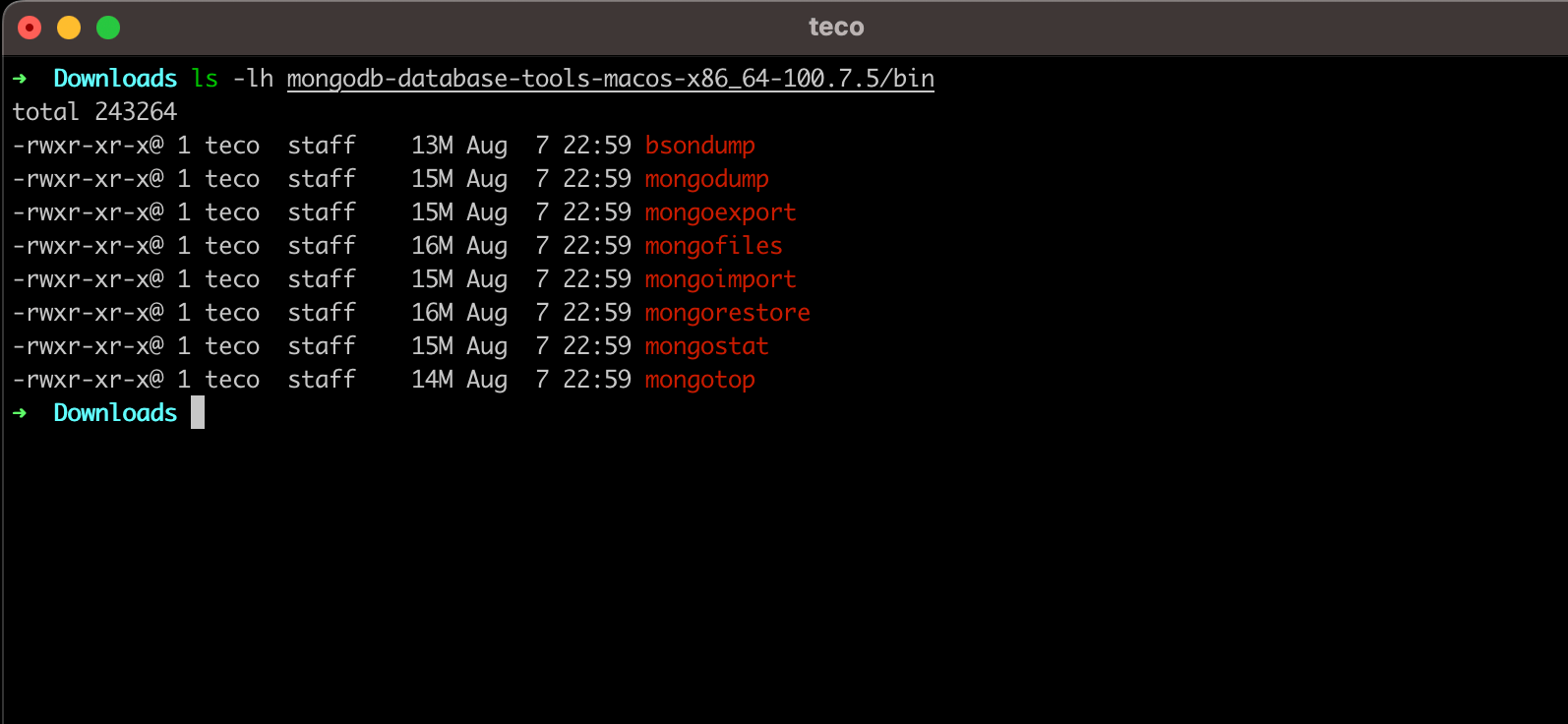
Move the "bin" folder in the home directory and rename the folder to "mongodb-tools" to easily recognize it later:
mv mongodb-database-tools-macos-x86_64-100.7.5/bin $HOME/mongodb-tools
rm -rf mongodb-database-tools-macos-x86_64-100.7.5
To run any MongoDB database tools, we must add the "$HOME/mongodb-tools" folder in the environment variable. On MacOS or Linux, you must edit the file. .bash_profile
, .profile
, .bashrc
or .zshrc
for those using ZSH like me.
Open the related file and append the line below:
export PATH=$PATH:$HOME/mongodb-tools
Save and close the file, and open a new terminal to check if MongoDB database tools commands are recognized.
mongoexport --version
mongoimport --version
mongodump --version
mongorestore --version
You will get the following output:
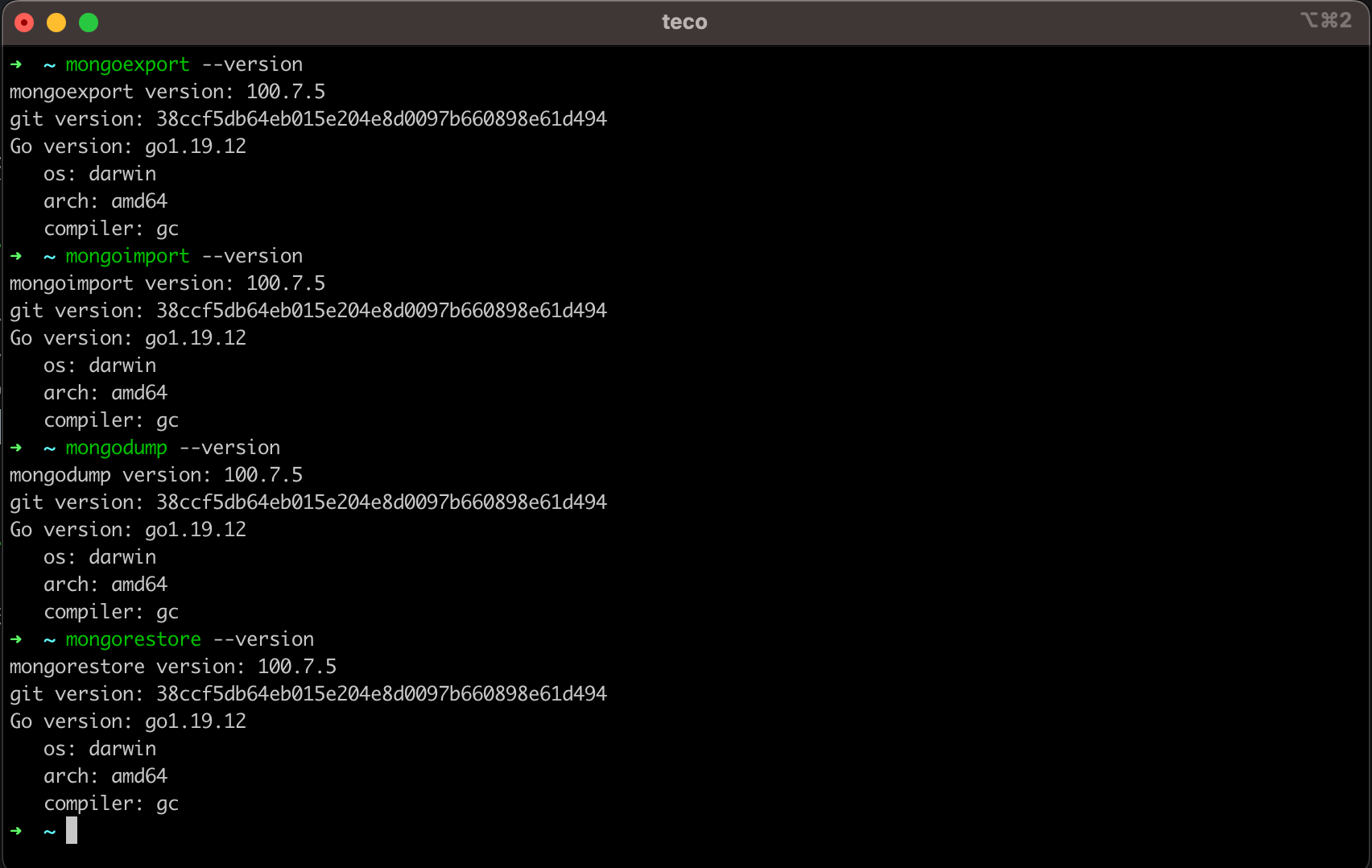
Export a collection of a MongoDB database
The database tool mongoexport
allows exporting a collection of the database. The syntax is the following:
mongoexport --uri "<mongo-uri>" --collection <collection-name> --out <filename>.<ext>
- The <mongo-uri> is the connection string to the MongoDB database that follows the format:
mongodb//:<db-user>:<db-password>@<mongo-host>:<mongo-port>/database?<connection-options>
. Check out the MongoDB documentation to learn more. - The <collection-name> is the collection's name inside the database you want to export.
- The <filename>.<ext> is the name of the filename and the extension to save on your computer disk; the possible extensions are JSON and CSV.
The data scraped from this tutorial I wrote about Web scraping in Node.js are stored in a MongoDB database on MongoDB Cloud.
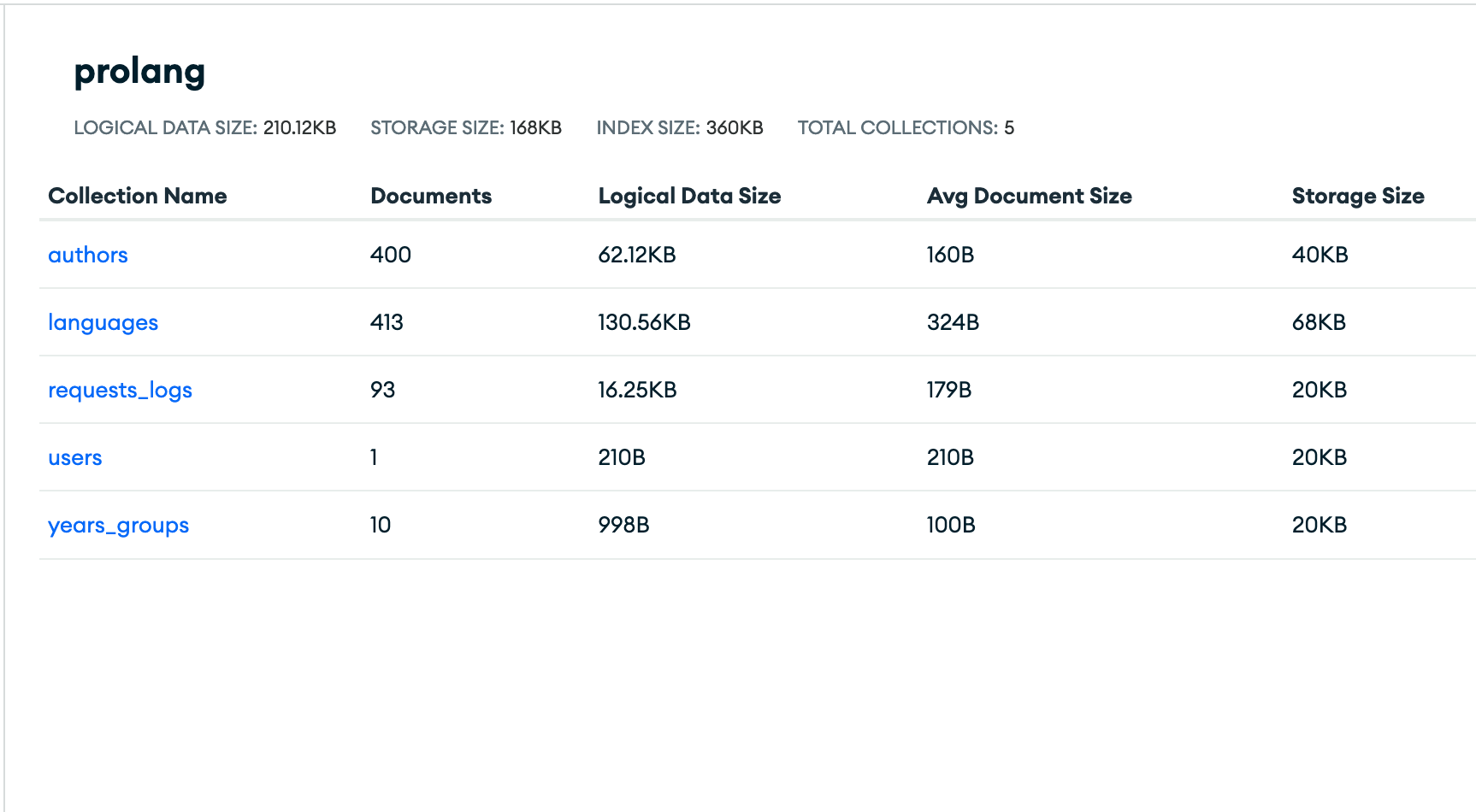
Let's export the collection "languages" into a file named "languages-list.json"
mongoexport --uri "mongodb+srv://teco:f4K3pAsSW0rD@cluster0.idihf.mongodb.net/prolang?retryWrites=true&w=majority" \
--collection languages \
--out languages-list.json
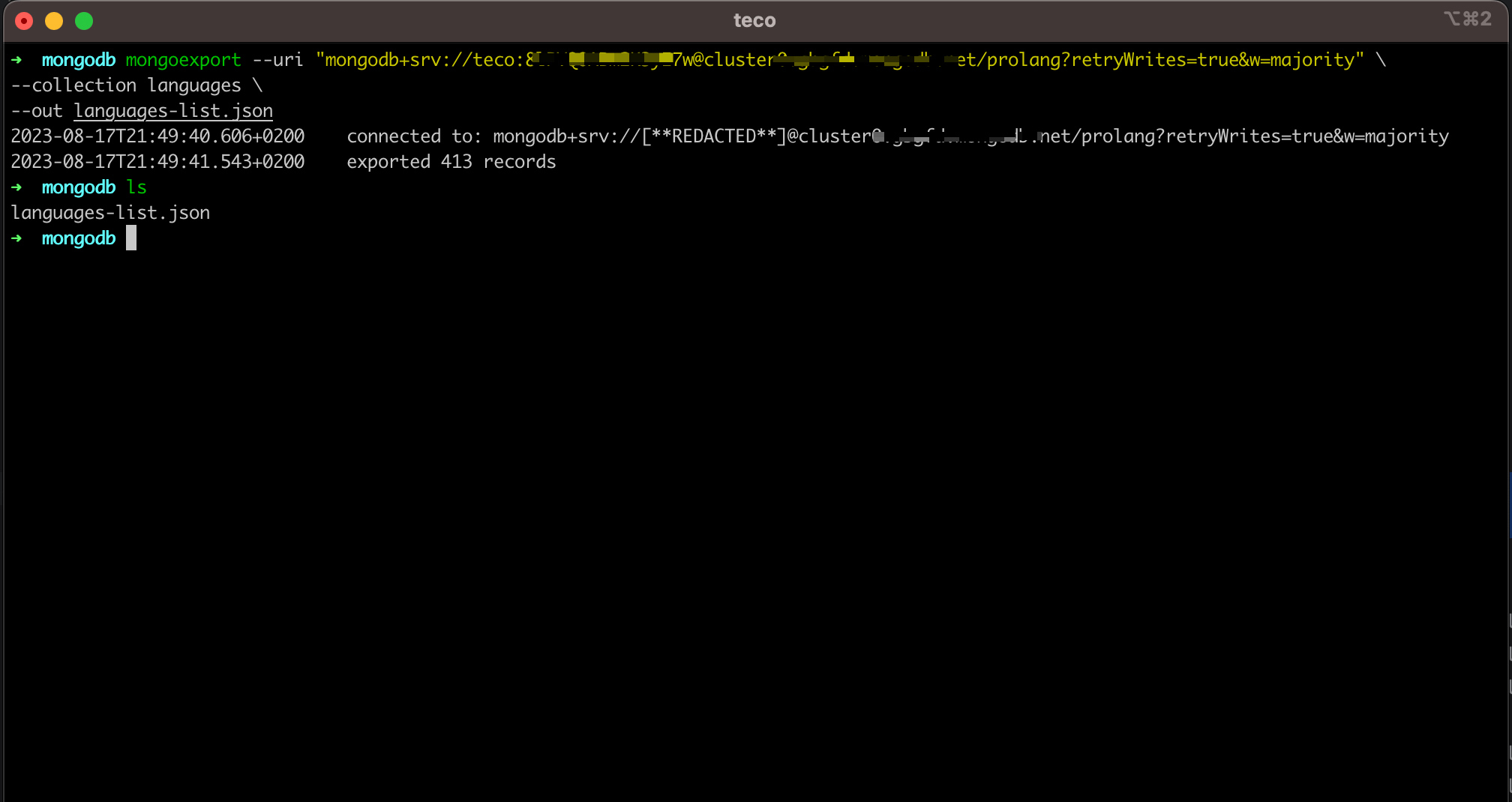
To export data from a MongoDB database collection in the CSV format, you must add the option --type
with the value "csv" and also the fields you want to export using the option --fields
:
mongoexport --uri "mongodb+srv://teco:f4K3pAsSW0rD@cluster0.idihf.mongodb.net/prolang?retryWrites=true&w=majority" \
--collection languages \
--type csv \
--fields _id,name,years \
--out languages-list.csv
Here, we only want to export the fields "_id", "name", and "years" from each MongoDB document in the collection "languages". If you want to export all the fields, you must explicitly declare them.
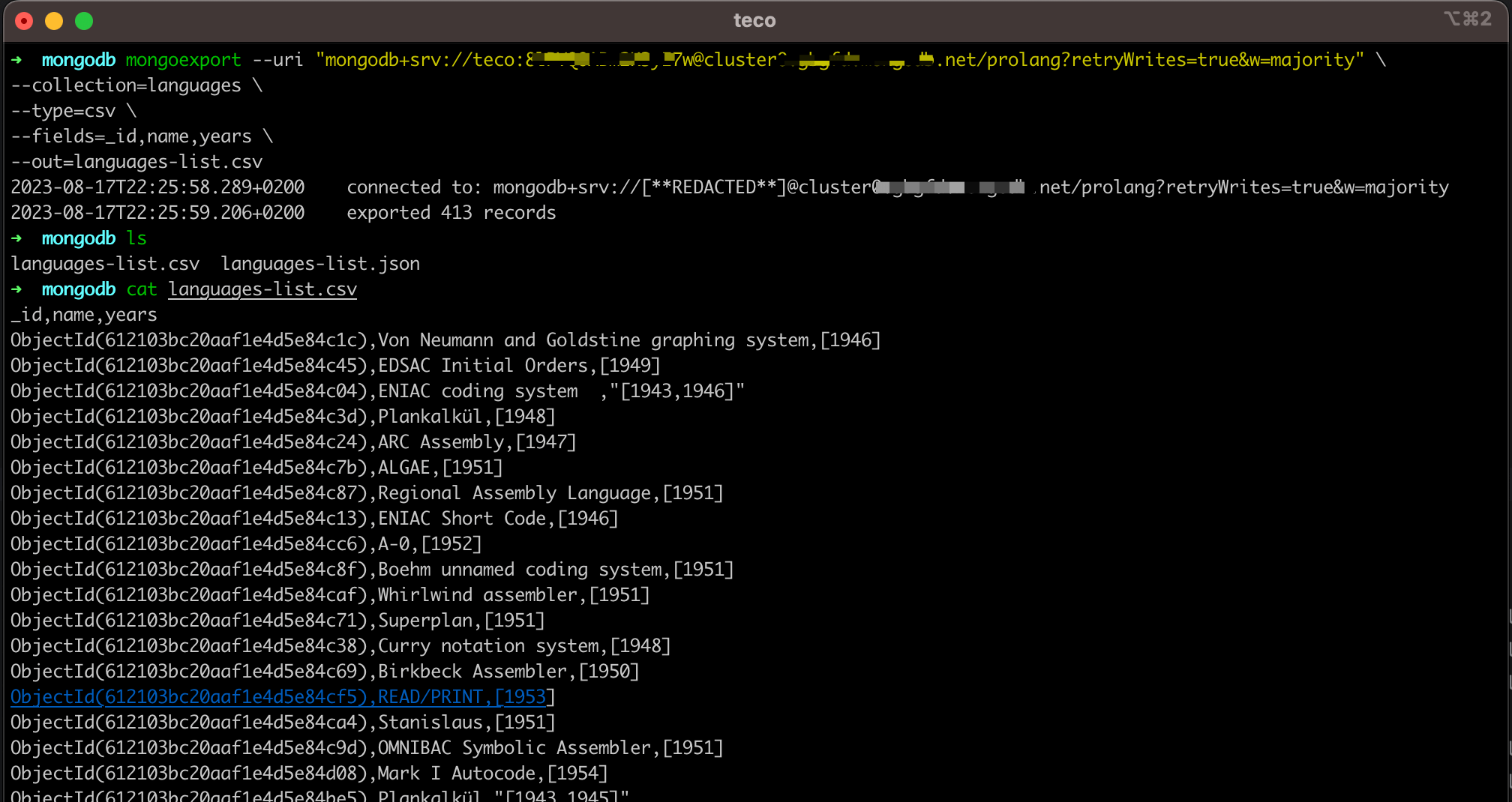
Import a collection of a MongoDB database
The database tool mongoimport
allows exporting a collection of the database. The syntax is the following:
mongoimport --uri "<mongo-uri>" --collection <collection-name> --file <filename>.<ext>
- The <collection-name> is the collection's name inside the database you want to import the data into.
- The <filename>.<ext> is the name of the filename and the extension on your computer disk containing the data to import; the possible extensions are JSON and CSV.
The code below imports the content of the file "languages-list.json" into a collection named "languages-list":
mongoimport --uri "mongodb+srv://teco:f4K3pAsSW0rD@cluster0.idihf.mongodb.net/prolang?retryWrites=true&w=majority" \
--collection languages-list \
--file languages-list.json
The collection is created if it doesn't exist in the database.
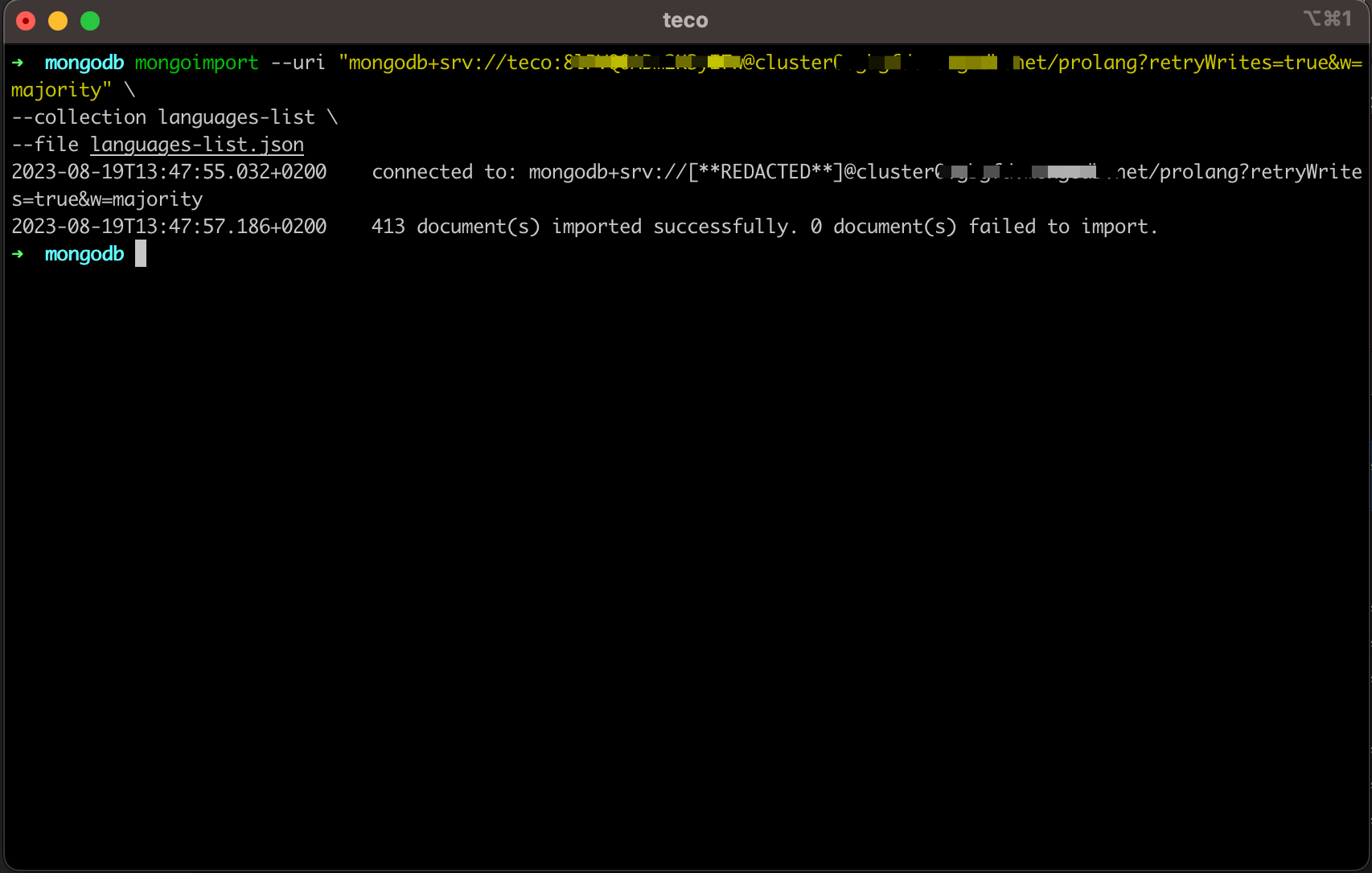
Import data into an existing MongoDB collection
The syntax is still the same as the previous import we did, but you can use the option "--mode" to define what happens if a document already exists in the collection:
- insert: Insert the document in the collection and throw an error if a duplicate document exists with the same "_id" or any unique fields in the document
- upsert: replace the document if it exists or create it otherwise.
- merge: when the document exists in the collection, the fields existing in the new document that doesn't exist in the old document will be imported.
- delete: a document found with the "_id" or any unique fields will be deleted; those not matching will be left as is.
By default, mongoimport
uses the field "_id" to match the document, but if you want to indicate other fields, use the option "--upsertFields".
It is useful when you import documents that don't have _id or when there are many fields unique field to identify a document (the email address for a person and the ISBN for a book).
The code below imports the content of the file "languages-list.json" into the collection named "languages-list", using the merge mode and the field "name" (the language's name) for the document matching:
mongoimport --uri "mongodb+srv://teco:f4K3pAsSW0rD@cluster0.idihf.mongodb.net/prolang?retryWrites=true&w=majority" \
--collection languages-list \
--file languages-list.json \
--mode merge \
--upsertFields name
Import data in a CSV file into a MongoDB collection
You must specify the options "--type" with the value "csv" and "--headerline" to indicate mongoimport
to infer the field's name from the first line of the CSV file.
The code below imports the content of the file "languages-list.csv" into the collection named "languages-list":
mongoimport --uri "mongodb+srv://teco:f4K3pAsSW0rD@cluster0.idihf.mongodb.net/prolang?retryWrites=true&w=majority" \
--collection languages-list \
--file languages-list.csv \
--type csv \
--heardline
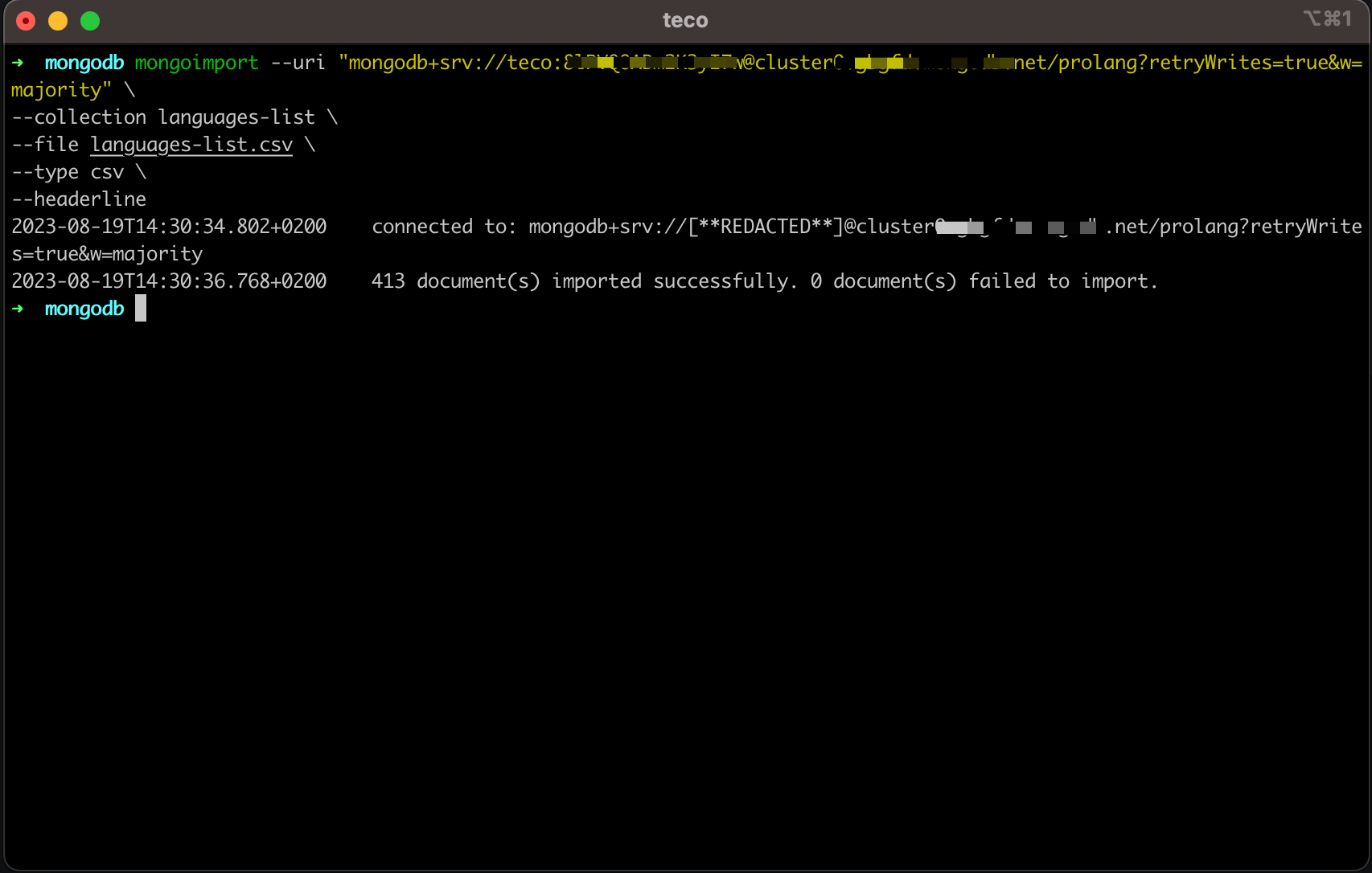
Backup a MongoDB database
Instead of exporting each collection individually, you export the whole database as a binary file using the database tool Mongodump.
The syntax is the following:
mongodump --uri "<mongo-uri>"
The code below backup the database on MongoDB Atlas to our computer:
mongodump --uri "mongodb+srv://teco:f4K3pAsSW0rD@cluster0.idihf.mongodb.net/prolang?retryWrites=true&w=majority"
Mongodump creates a folder "dump/<database-name>" in the current directory.
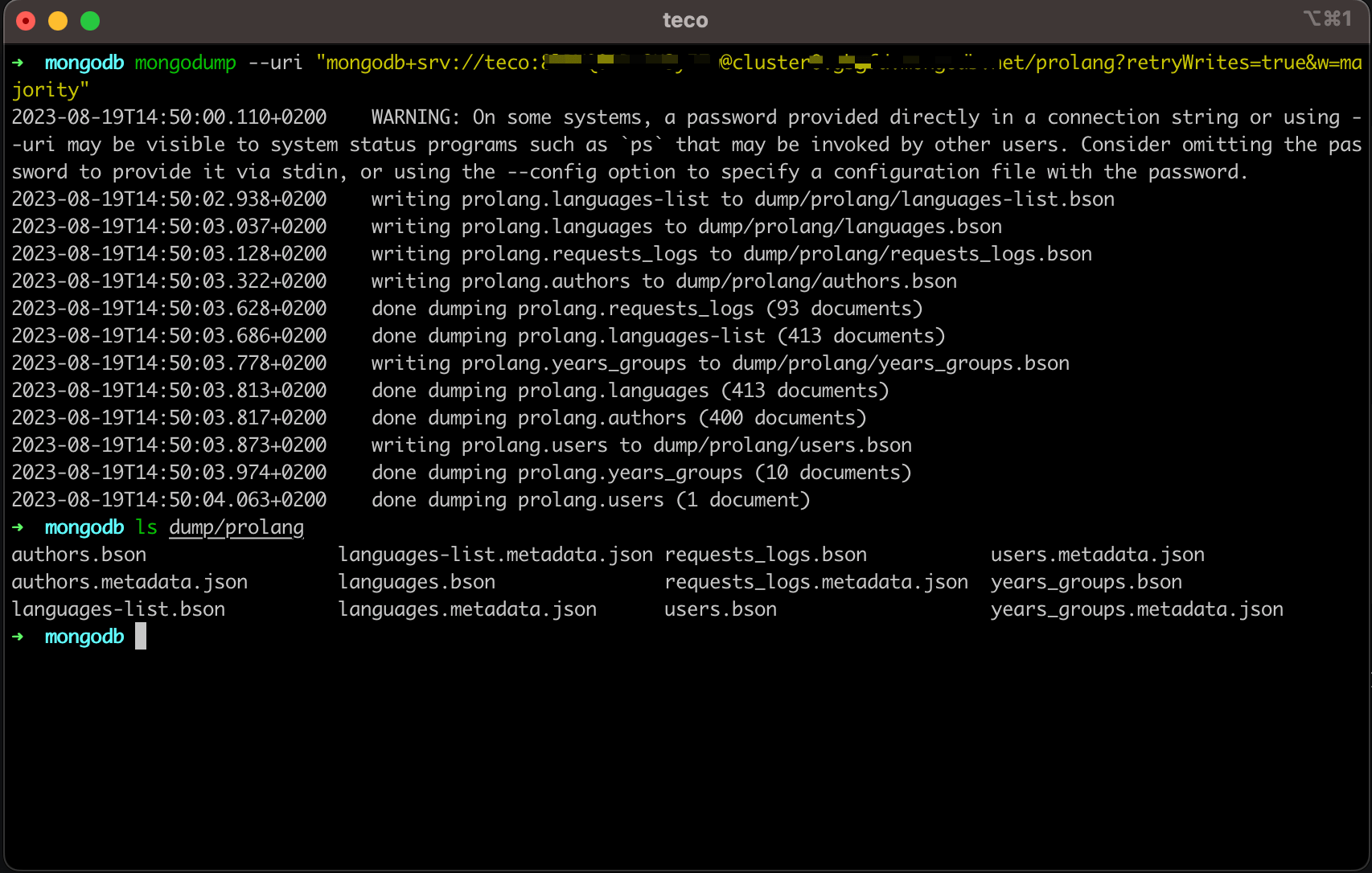
You can also provide the URI for the MongoDB replicat set, and Mongodump will figure out how to perform the backup.
Restore a MongoDB database
The syntax to restore a database from a dump folder is the following:
mongorestore --uri "<mongo-uri>" <dump-folder>
The code below restores the database from the dump folder located on our computer into a database named "prolang-copy" on MongoDB Atlas:
mongorestore --uri "mongodb+srv://teco:8lPVQOABm2K3yI7w@cluster0.gbgfd.mongodb.net/prolang-copy?retryWrites=true&w=majority" \
./dump/prolang
If the database doesn't exist, it will be created.
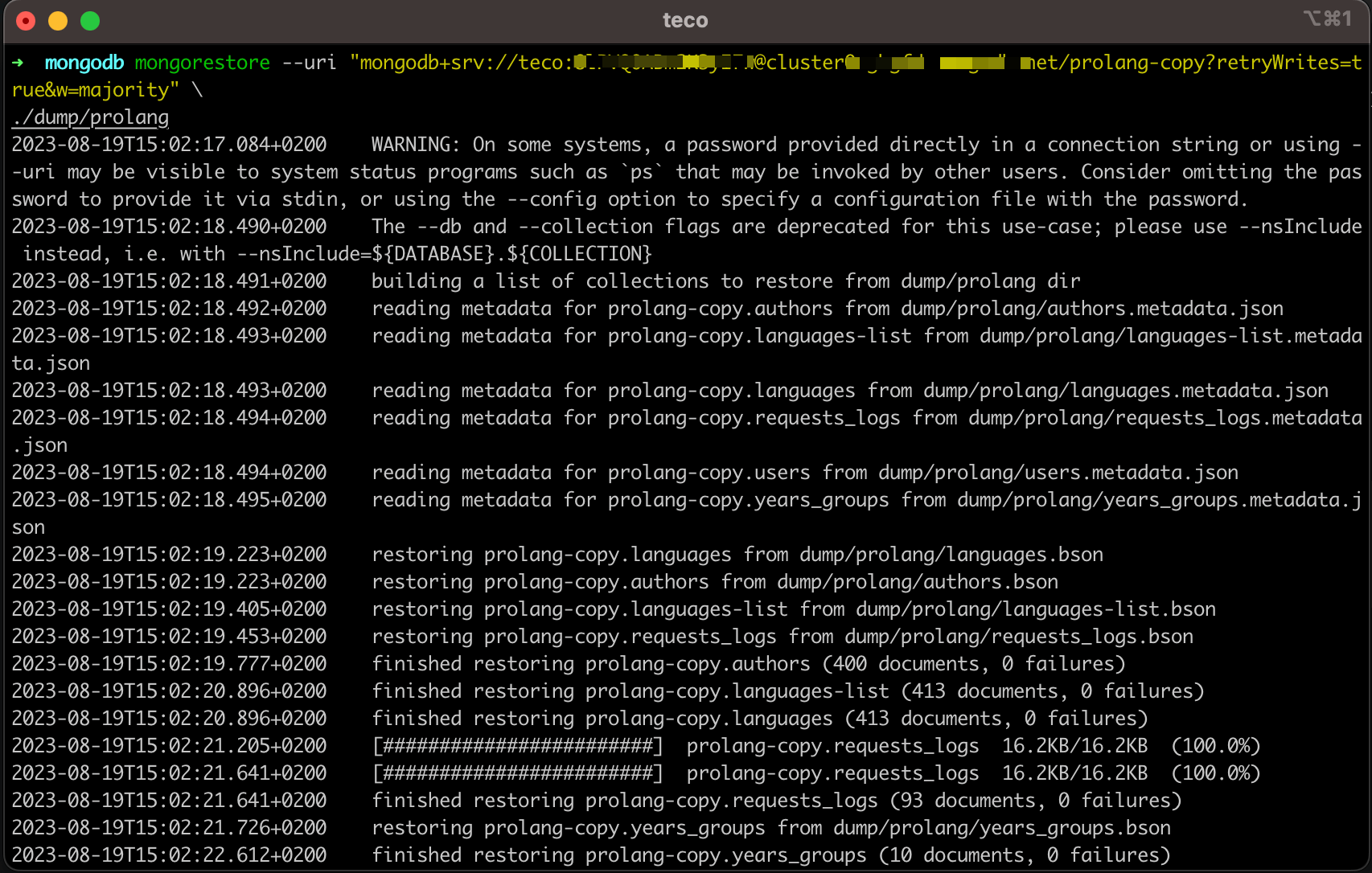
Wrap up
The MongoDB database tools allow easy backup and restore of your database. We saw how to use mongoexport
, mongoimport
, mongodump
, and mongorestore
with the most essential options.
To learn more about the capability of each tool, check out the respective links below:
- Mongo export documentation
- Mongo import documentation
- Mongo Dump documentation
- Mongo Restore documentation
Follow me on Twitter or subscribe to my newsletter to avoid missing the upcoming posts and the tips and tricks I occasionally share.