Get the user IP address in a Node.js application with Express
When building a Node.js application with the framework Express, retrieving the user IP address can be used to implement interesting features such as:
- Geolocate the user country to adapt the content to serve
- Build a Purchase Pricing Parity (PPP)
- Implement IP-based rate limiting
- Any feature where you need the IP address.
This post will show how to retrieve the user's IP address.
Prerequisites
To follow this post, you will need to install this tool on your computer:
- Node.js 16 or higher - download link
- A Node.js package manager such as NPM or Yarn (I will use Yarn)
Set up the project
We will use the Node.js starter project we built in this post; the project contains a branch express
that comes with the framework Express. We will use it further in this tutorial.
Run the commands below:
git clone https://github.com/tericcabrel/node-ts-starter.git -b express node-ip-address
cd node-ip-address
cp .env.example .env
# Update the environment variable if needed
nano .env
yarn install
yarn start
Open your browser and navigate to the URL http://localhost:4500.
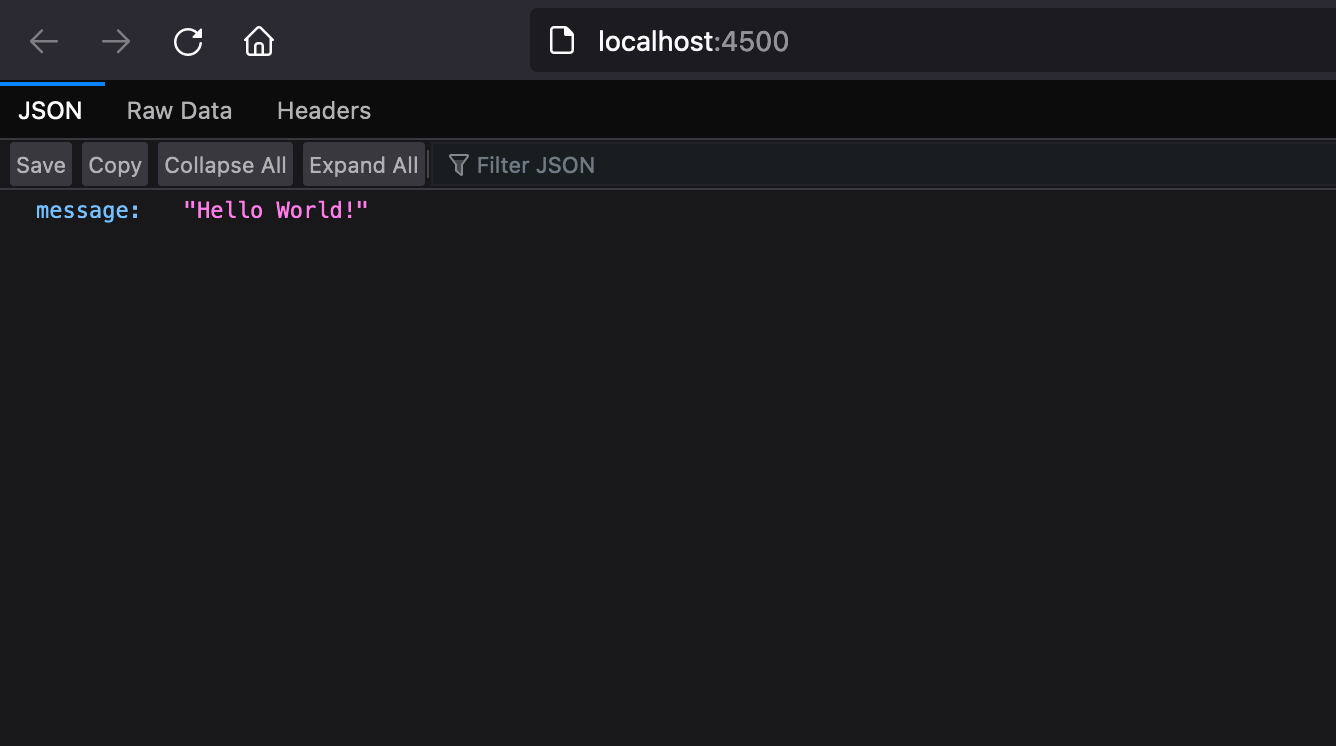
In the file src/index.ts
, the code below is responsible for displaying a creating a route with the path /
that returns a JSON message.
app.get('/', (req, res) => {
return res.json({ message: 'Hello World!' });
});
The app.get()
callback's function has two arguments req
and res
corresponding to the Express request and response object.
The Express request object contains information about the request sent from the client, such as the headers, the body, the path, and the client's IP address.
Get the IP address
The Express request object contains the property ip
containing the IP address in the IPv6 format. We will add a new route /ipv4
that will return the IP address.
Let's update the code of the file src/index.ts
by adding the code below:
app.get('/ipv4', (req, res) => {
const ipAddress = req.ip;
return res.json({ message: `Hello! Your IP address is: ${ipAddress}` });
});
Re-run the application and refresh the browser and navigate to http://localhost:4500/ipv4.
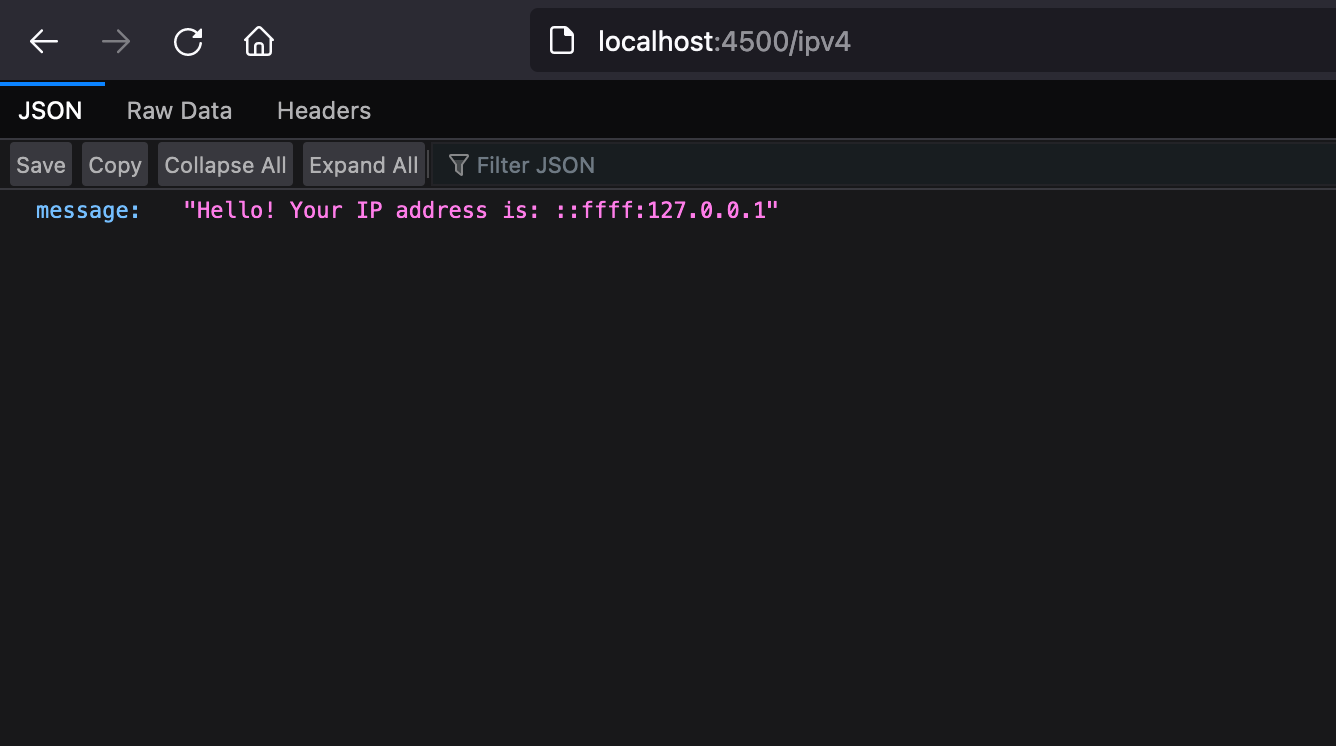
Since we are running the Node.js application locally, the IP address corresponds to the local computer displayed in the IPv6 format. Further, we will see the difference when running it on a remote server.
Forward the IP address to the Reverse proxy
I deployed this application on a virtual server accessible through the subdomain nodeip.tericcabrel.com using Nginx as a reverse proxy.
I wrote the blog post below to help you if you want to learn to do that.
Below is the Nginx configuration for this Node.js application:
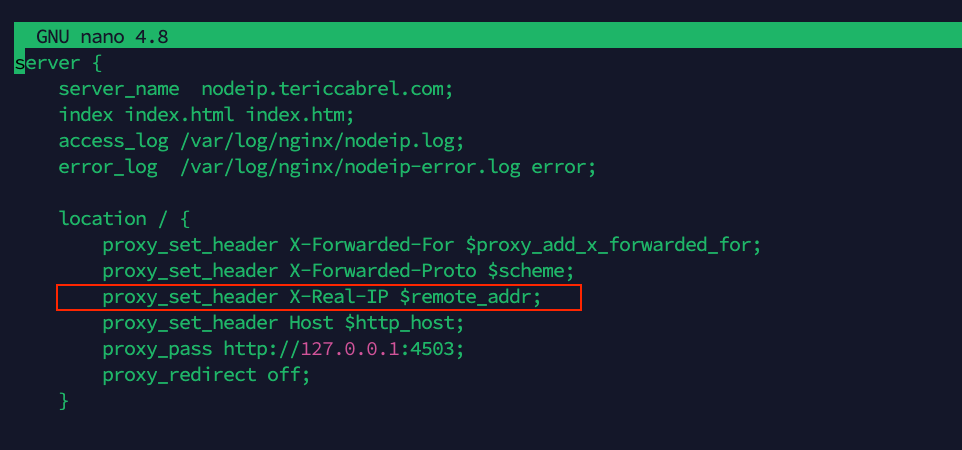
The configuration line proxy_set_header X-Real-IP $remote_addr;
is responsible for forwarding the real user IP address to the Node.js application. Always ensure this configuration is present.
Let's browse the application online to see the result:
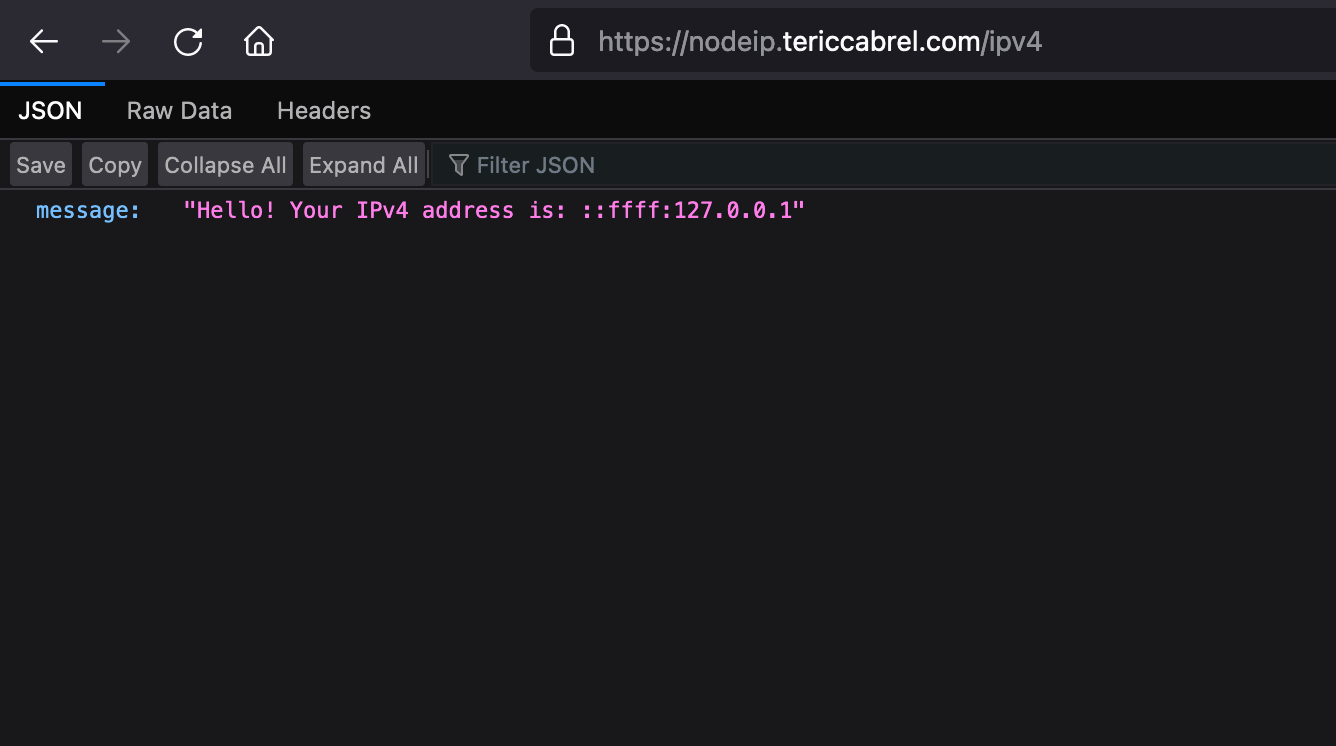
We still don't get the real user IP address because Express doesn't trust information forwarded from a reverse proxy, and thus the configuration is disabled by default. We must update our code to add the line below:
app.set('trust proxy', true);
This configuration enabled trust from the reverse proxy. Let's redeploy the application on the server to see the result.
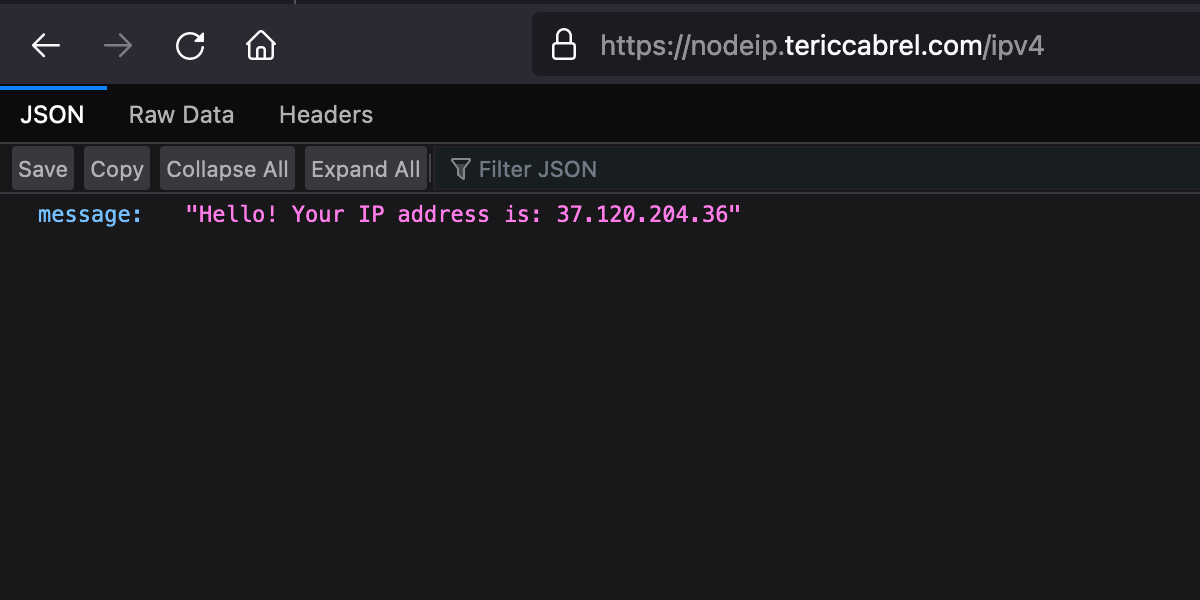
Of course, I used a VPN, so don't try to locate me 😝
Wrap up
Getting the user IP address allows building interesting features from your Node.js application. Here are the takeaways:
- The IP address is retrieved from the Express request object
- Ensure to forward the real user IP address in the reverse proxy configuration
- Enable Express configuration to trust the reverse proxy.
You can find the code source on the GitHub repository.
Follow me on Twitter or subscribe to my newsletter to avoid missing the upcoming posts and the tips and tricks I occasionally share.